Java基础语法
Java是一门通用性编程语言,其特征包括但不限于面对对象、跨平台、多线程、高性能等等.Java的最大的特点是跨平台,利用及其稳定的Java虚拟机(Java Virtual Machine)同样的源代码可能在任何平台运行。这不仅仅提高了软件的可移植性,也简化了开发者的工作。
Java是一门设计优良的编程语言,它是一门完全的面向对象编程语言,面向对象编程是一种重要的编程思想,对于构建复杂的系统有很大的积极作用.
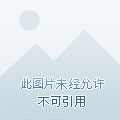
Java 介绍和环境配置
Java最早是由SUN公司开发的,最早被命名为Oak,最初主要针对于小型家电的嵌入器应用。后来互联网崛起,SUN改造了Oak,在1995以Java的名称正式发布,随着互联网的快速发展,Java也成了最受欢迎的语言。
Java分出了三个不同版本:Java SE (Standard Edition),Java EE (Enterprise Edition) ,和Java ME (Micro Edition).
- Java SE (Standard Edition) 标准版本,其他包含了JVM和常用的库.
- Java EE (Enterprise Edition)是企业版本,在Java SE的基础上加了更多的库和API,提供了Web应用、数据库和消息服务等等的库,方便了企业级应用的开发.
- Java ME (Micro Edition) 嵌入式设备Java开发语言平台.
JVM (Java Virtual Machine)
JVM使你的Java代码在不同的计算机平台上能够顺利运行.一串Java代码在计算机上运行的步骤是:
- Java编译器将 Java代码编译成Java字节码
- JVM将Java字节码转换成计算机平台支持的机器码(计算机的CPU直接执行的一组指令)
- CPU执行机器码
JRE(Java Runtime Environment)
RE(Java运行时环境)是一个软件包,提供Java类库,Java虚拟机(JVM)和运行Java应用程序所需的其他组件。
如果你只需要运行Java代码而不需要开发Java程序,则你只需下载JRE就够了.
JDK(Java Development Kit)
JDK(Java开发套件)是使用Java开发应用程序所需的软件开发套件。当您下载JDK时,还将同时下载JRE。JDK除了包含JRE,还包含许多开发工具(编译器,JavaDoc,Java Debugger等)。
如果你需要进行Java程序开发,则应该下载JDK.
JDK、JRE、JVM的关系如下图:
下载安装Java开发环境
去oracle官网下载最新的 Java SE的 JDK.
下载完JDK后,我们需要设置JAVA的环境变量,这样系统运行Java代码时,才能从正确的路径调用程序来运行代码。
MAC
使用Mac的朋友可以使用以下的步骤来设置环境变量:
将下载好的安装包解压缩后,记住安装目录的路径,然后在~/.bach_profile中设置新的环境变量:
1 | export JAVA_HOME=/Users/haozheng/Java/jdk-13.0.1.jdk/Contents/Home |
在上面的步骤中,我们将JAVA_HOME的bin(binary)目录添加到PATH是为了在任意文件夹下都可以运行java。
最后在Terminal中运行 java -version 如果出现以下信息就代表Java安装成功了!
1 | $ java -version |
Windows
Windows平台在安装完成后,也是需要将 jdk 的bin目录添加到 Path
中.
运行Java 代码
写完后的Java代码运行示意图如:
源代码被Java编译器转化成字节码(Byte Code),然后通过JVM这个虚拟机,字节码就能在各个平台上运行。
首先我们使用javac编译器将源代码转化成字节码,在Main.java的目录下执行命令 javac Main.java(源代码):
1 | $ javac Main.java |
如果没有任何的语法错误,那么当前目录中就会多出一个 Main.class 文件(字节码):
1 | $ ls |
接下来我们就能使用 java Main 来运行程序:
1 | $ java Main |
变量和内置数据类型
变量
变量是内存中用于存储数据的位置。为了指示存储区域,应该为每个变量赋予唯一的名称(标识符)。
创建变量的语法:以数据类型开始, 空格后面是变量的名字,然后可以紧跟着是数值,最后必须以分号( ; )结尾.
1 | int speedLimit = 80; |
speedLimit
是int
数据类型的变量,并分配了值80.
您可以在不分配值的情况下声明变量,以后可以根据需要存储值。
1 | int speedLimit; |
变量的值可以在程序中更改,因此名称为“变量”。
1 | int speedLimit = 80; |
Java是一种静态类型的语言。这意味着必须先声明所有变量,然后才能使用它们。
同样,您不能在同一范围内更改Java中变量的数据类型。
变量命名规则
Java编程语言具有自己的一组变量命名规则和约定
- Java中的变量区分大小写.
- 变量的名称是Unicode字母和数字的序列。它可以以字母,$或_开头。但是习惯上以字母开头。同时变量名不能使用空格.
- 创建变量时,请选择一个有意义的名称。例如:
scope
、num
比s
、n
更好理解. - 如果选择一个单词的变量名,请使用所有小写字母。
- 如果选择具有多个单词的变量名,请对第一个单词使用所有小写字母,并在每个后续单词中使用大写字母。
变量类型
Java语言中有四种变量类型:
- 实例变量(非静态字段)
- 类变量(静态字段)
- 局部变量
- 参数
内置数据类型
Java中有8种基本内置数据类型,这些数据类型的大小和作用各不相同:
数据类型 | 数据大小 | 具体描述 |
---|---|---|
byte | 1 byte | -128到127之间的整数 |
short | 2 bytes | -32,768到32,767之间的整数 |
int | 4 bytes | 整数(-2,147,483,648 ~ 2,147,483,647) |
long | 8 bytes | 长整数(-9,223,372,036,854,775,808 ~ 9,223,372,036,854,775,807) |
float | 4 bytes | 浮点数(小数后6到7位) |
double | 8 bytes | 浮点数(小数后15位) |
boolean | 1 bit | true 或 false(正或否) |
char | 2 bytes | 单个字符或字母(ASCII数值) |
boolean
- 布尔数据类型具有两个可能的值,即true或false.
- 默认值:false.
- 它们通常用于 true/false 条件.
1 | class BooleanExample { |
输出:
1 | true |
byte
- byte类型的值可以从-128到127(8位带符号二进制补码整数).
- 如果可以确定变量的值在[-128,127]之内,则可以使用它代替int或其他整数数据类型来节省内存.
- 默认值:0
1 | class ByteExample { |
输出:
1 | 124 |
short
- short类型的值可以从-32768到32767(16位带符号二进制补码整数).
- 如果可以确定变量的值在[-32768,32767]之内,则可以使用它代替其他整数数据类型来节省内存.
- 默认值:0
1 | class ShortExample { |
输出:
1 | -200 |
int
- int数据类型的值可以从-2,147,483,648 ~ 2,147,483,647(32位带符号二进制补码整数)
- 如果使用Java 8或更高版本,则可以使用无符号32位整数,最小值为0。
- 默认值:0
1 | class IntExample { |
输出:
1 | -4250000 |
long
- 长数据类型的值可以从(-9,223,372,036,854,775,808 ~ 9,223,372,036,854,775,807)(64位带符号的二进制补码整数)
- 如果您使用的是Java 8或更高版本,则可以使用最小值为0的无符号64位整数
- 默认值:0
1 | class LongExample { |
输出:
1 | -42332200000 |
double
- double数据类型是双精度64位浮点
- 切勿将其用于诸如货币之类的精确值
- 默认值:0.0(0.0d)
1 | class DoubleExample { |
输出:
1 | -42.3 |
float
- float数据类型是单精度32位浮点.
- 切勿将其用于诸如货币之类的精确值.
- 默认值:0.0(0.0f)
1 | class FloatExample { |
输出:
1 | -42.3 |
如果需要告诉编译器变量是单精度,应该在数值后面加一个f
.
char
- 这是16位Unicode字符.
- char数据类型的最小值为
'\u0000'
(0),char数据类型的最大值为'\uffff'
. - 默认值:
'\u0000'
1 | class CharExample { |
输出:
1 | Q |
您得到输出Q,因为Q的Unicode值为'\u0051'
1 | class CharExample { |
输出:
1 | 9 |
当您打印letter1时,您会得到9,因为letter1被分配了字符’9’。 当打印letter2时,得到A的原因是ASCII值’A’为65。这是因为java编译器将字符视为整数类型.
运算符
赋值运算符
赋值运算符是给变量
赋值
1 | int age |
赋值运算符将其右侧的值分配给其左侧的变量。在此,使用=运算符将5分配给age。
1 | class AssignmentOperator { |
算数运算符
算术运算符用于执行数学运算,例如加法,减法,乘法等。
Operator | Meaning |
---|---|
+ | 加法(也用于字符串连接) |
- | 减法运算符 |
* | 乘法运算符 |
/ | 除法运算符 |
% | 求余运算符 |
1 | class ArithmeticOperator { |
输出:
1 | number1 + number2 = 16.0 |
add 连接字符串
1 | class ArithmeticOperator { |
一元运算符
一元运算符仅对一个操作数执行运算。
Operator | Meaning |
---|---|
+ |
一元加号(由于数字为正数而不使用它,因此无需使用) |
- |
一元减:反转表达式的符号 |
++ |
增量运算符:将值增加1 |
-- |
递减运算符:将值减1 |
! |
逻辑补码运算符:反转布尔值 |
1 | class UnaryOperator { |
输出:
1 | +number = 5.2 |
自增或自减运算符
您也可以在Java中同时使用++和-运算符作为前缀和后缀。 ++运算符将值增加1,而-运算符将值减少1。
1 | int myInt = 5; |
等于与关系运算符
等于和关系运算符确定两个操作数之间的关系。它检查操作数是否大于,小于,等于,不等于等。根据关系,它被评估为true或false。
Operator | Description | Example |
---|---|---|
== |
等于 | 5 == 3 is evaluated to false |
!= |
不等于 | 5 != 3 is evaluated to true |
> |
大于 | 5 > 3 is evaluated to true |
< |
小于 | 5 < 3 is evaluated to false |
>= |
大于等于 | 5 >= 5 is evaluated to true |
<= |
小于等于 | 5 <= 5 is evaluated to true |
1 | class RelationalOperator { |
输出:
1 | number2 is greater than number1. |
instanceof 操作符
除了关系运算符外,还有一个类型比较运算符instanceof,它将对象与指定类型进行比较。例如,
1 | class instanceofOperator { |
逻辑运算符
逻辑运算符|| (条件OR)和&&(条件AND)对布尔表达式进行运算
1 | class LogicalOperator { |
三元运算符
1 | variable = Expression ? expression1 : expression2 |
1 | class ConditionalOperator { |
位运算符
Operator | Description |
---|---|
~ |
按位补码 |
<< |
左移 |
>> |
右移 |
>>> |
无符号右移 |
& |
位与 |
^ |
位异或 |
` | ` |
Java 基础输入和输出
输出
Java标准输出方法有:
1 | System.out.println() // 输出数据,自动换行 |
System
是个 class,out
是一个公用的静态字段,用来接受需要输出的数据.
1 | class Output { |
输入
Java提供了多种输入方式给用户使用. 这里面介绍Scanner
类.
使用Scanner
需要引入Java包java.util.Scanner
.
import java.util.Scanner
1 | import java.util.Scanner; |
支持获取 浮点、字符串类型值
1 | import java.util.Scanner; |
输出:
1 | /* |
Java表达式、语句、代码块
表达式
Java表达式有:变量、运算符、字面量和方法调用组成.
1 | int score; |
语句
在Java中,每个一个语句是最小执行单元.
1 | int score = 9*5; |
表达式语句
我们可以通过;
将表达式来将表达式转换为语句.
1 | // 表达式 |
声明语句
Java中声明语句,是声明一个变量:
1 | Double tax = 9.5; |
Java代码块
1 | class Main { |
代码块是用大括号{}括起来的一组语句(零个或多个).
注释
Java提供两种注释方式: 单行和多行的.
1 | /* This is an example of multi-line comment. |
数组
声明数组
1 | String[] array = new String[100]; |
上面声明了长度为100的字符串类型数组.
初始化数组
1 | //declare and initialize and array |
1 | class Main { |
遍历数组
Java中常用 for
和for-each
遍历数组
1 | class Main { |
1 | class Main { |
声明多个数组
1 | double[][] matrix = { |
数组复制
直接赋值
1 | class Main { |
直接用=
赋值,当修改一个变量的数据时,是会影响到引用的变量. 不建议使用该方法复制数组
遍历数组复制数组
1 | import java.util.Arrays; |
在上面的示例中,我们使用了for循环迭代源数组的每个元素。在每次迭代中,我们都将元素从源数组复制到目标数组。
使用arraycopy()
方法
在Java中,System类包含一个名为arraycopy()
的方法来复制数组。与上述两种方法相比,此方法是复制数组的更好方法。
arraycopy()
方法允许您将源数组的指定部分复制到目标数组。
1 | arraycopy(Object src, int srcPos,Object dest, int destPos, int length) |
- src 需要copy的数组
- srcPos 复制开始数组下标
- dest 目标数组,将从源中复制元素
- destPos 目标数组中的起始位置(索引)
- length 复制元素数量
1 | // To use Arrays.toString() method |
使用copyOfRange()
方法
1 | // To use toString() and copyOfRange() method |
int[] destination1 = Arrays.copyOfRange(source,index,length)
将源数组复制给目标 数组变量
复制二维数组
用System.arraycopy()
复制二维数组
1 | import java.util.Arrays; |
流程控制
if-else
if-else
常见使用方式有四种:
1 | if(condition) { |
condition
和otherCondition
是一个 boolean表达式. 它会返回true
或false
.
condition
结果如果是true
,则执行中括号内的内容.condition
结果如果是false
,则跳过中括号的内容.
if-else
支持互相嵌套.
switch
switch
语法是在一组可供执行的方案中,确定符合条件的方案进行执行.
语法:
1 | switch (expression) { |
expression
将执行一次,得到的结果将和 case
中声明的 value
进行匹配.如果匹配成功,则执行 case
后面的代码块·.
如果case
中未能匹配成功,则执行default
中的代码块.
switch
执行流程图:
代码demo:
1 | // Java Program to check the size |
Java for Loop
根据条件重复执行相同代码的语法在java中常见的有3种,for
循环是其中之一.
1 | for (initialExpression; testExpression; updateExpression) { |
initialExpression
- 初始化或声明变量,只执行一次.testExpression
- 执行条件判断,返回值是boolean
类型,如果返回是true
则执行for
循环中括号内的代码块.updateExpression
- 更新initialExpression
中声明的变量值.for
循环中括号内的代码块执行完成后,则再执行testExpression
直到其值返回false
结束for
循环.
for
循环执行流程:
for
循环例子:
1 | class Main { |
Java for-each Loop
for-each
是 另外一种遍历数组的方式.使用了for-each循环来逐一打印数字数组的每个元素.
语法:
1 | for(dataType item : array) { |
array
- 数组或集合.item
- 数组或集合的每一项分配的变量.dataType
- 数组或集合的数据类型.
for-each
代码例子
1 | // Calculate the sum of all elements of an array |
Java while and do…while Loop
Java while loop
while
loop是执行重复代码直到判断条件为false
1 | while (testExpression) { |
textExpression
- 执行条件,返回boolean
值类型.如果结果是true
则执行while
中括号内的代码块.如果结果是false
则跳过while
循环.
while
loop 执行流程:
代码例子:
1 | // Java program to find the sum of positive numbers |
Java do…while loop
do...while
和while
很类似,但不同的是 do...while
会先执行一次中括号内的代码块再进行条件判断.
语法:
1 | do { |
代码例子:
1 | // Java Program to display numbers from 1 to 5 |
Java break Statement
Java中的break
语句立即终止循环,程序的控制移至循环后的下一条语句。
例子:
代码例子:
1 | import java.util.Scanner; |
常用使用场景:
Java continue Statement
continue
用于在数组/集合中遍历跳过本次循环.
使用场景:
作者: Fynn
链接: https://fynn90.github.io/2020/10/19/Java%E5%9F%BA%E7%A1%80%E8%AF%AD%E6%B3%95/
本文采用知识共享署名-非商业性使用 4.0 国际许可协议进行许可